快速入门#
备注
有关安装说明,请参见此处。
在深入研究核心 API 之前,让我们从一个简单的例子开始,两个代理创建特斯拉和英伟达的股票收益图。
我们首先定义代理类及其各自的消息处理程序。
我们创建两个代理类:Assistant
和 Executor
。Assistant
代理编写代码,Executor
代理执行代码。
我们还创建一个 Message
数据类,它定义了在代理之间传递的消息。
本例中生成的代码在 Docker 容器内运行。在运行示例之前,请确保已安装 Docker 并运行。本地代码执行是可用的(LocalCommandLineCodeExecutor
),但由于在本地环境中运行 LLM 生成的代码存在风险,因此不推荐使用。
from dataclasses import dataclass
from typing import List
from autogen_core.base import MessageContext
from autogen_core.components import DefaultTopicId, RoutedAgent, default_subscription, message_handler
from autogen_core.components.code_executor import CodeExecutor, extract_markdown_code_blocks
from autogen_core.components.models import (
AssistantMessage,
ChatCompletionClient,
LLMMessage,
SystemMessage,
UserMessage,
)
@dataclass
class Message:
content: str
@default_subscription
class Assistant(RoutedAgent):
def __init__(self, model_client: ChatCompletionClient) -> None:
super().__init__("An assistant agent.")
self._model_client = model_client
self._chat_history: List[LLMMessage] = [
SystemMessage(
content="""Write Python script in markdown block, and it will be executed.
Always save figures to file in the current directory. Do not use plt.show()""",
)
]
@message_handler
async def handle_message(self, message: Message, ctx: MessageContext) -> None:
self._chat_history.append(UserMessage(content=message.content, source="user"))
result = await self._model_client.create(self._chat_history)
print(f"\n{'-'*80}\nAssistant:\n{result.content}")
self._chat_history.append(AssistantMessage(content=result.content, source="assistant")) # type: ignore
await self.publish_message(Message(content=result.content), DefaultTopicId()) # type: ignore
@default_subscription
class Executor(RoutedAgent):
def __init__(self, code_executor: CodeExecutor) -> None:
super().__init__("An executor agent.")
self._code_executor = code_executor
@message_handler
async def handle_message(self, message: Message, ctx: MessageContext) -> None:
code_blocks = extract_markdown_code_blocks(message.content)
if code_blocks:
result = await self._code_executor.execute_code_blocks(
code_blocks, cancellation_token=ctx.cancellation_token
)
print(f"\n{'-'*80}\nExecutor:\n{result.output}")
await self.publish_message(Message(content=result.output), DefaultTopicId())
您可能已经注意到,代理的逻辑,无论是使用模型还是代码执行器,都与消息如何传递完全解耦。这是核心理念:框架提供通信基础设施,而代理负责自己的逻辑。我们将通信基础设施称为Agent Runtime。
代理运行时是这个框架的一个关键概念。除了传递消息外,它还管理代理的生命周期。 因此,代理的创建由运行时处理。
以下代码展示了如何使用 SingleThreadedAgentRuntime
(一个本地嵌入式代理运行时实现)来注册和运行代理。
import tempfile
from autogen_core.application import SingleThreadedAgentRuntime
from autogen_ext.code_executors import DockerCommandLineCodeExecutor
from autogen_ext.models import OpenAIChatCompletionClient
work_dir = tempfile.mkdtemp()
# Create an local embedded runtime.
runtime = SingleThreadedAgentRuntime()
async with DockerCommandLineCodeExecutor(work_dir=work_dir) as executor: # type: ignore[syntax]
# Register the assistant and executor agents by providing
# their agent types, the factory functions for creating instance and subscriptions.
await Assistant.register(
runtime,
"assistant",
lambda: Assistant(
OpenAIChatCompletionClient(
model="gpt-4o",
api_key="sk-"
)
),
)
await Executor.register(runtime, "executor", lambda: Executor(executor))
# Start the runtime and publish a message to the assistant.
runtime.start()
await runtime.publish_message(
Message("Create a plot of NVIDA vs TSLA stock returns YTD from 2024-01-01."), DefaultTopicId()
)
await runtime.stop_when_idle()
--------------------------------------------------------------------------------
Assistant:
To create a plot of NVIDIA vs TSLA stock returns, we'll need to fetch the stock data from a financial data provider such as Yahoo Finance using the `yfinance` library. Then, we'll compute the year-to-date (YTD) returns for both stocks and plot them.
Below is the Python script to achieve this:
```python
import yfinance as yf
import matplotlib.pyplot as plt
import pandas as pd
# Define the stock symbols
stocks = ['NVDA', 'TSLA']
# Define the start and end dates
start_date = '2024-01-01'
end_date = pd.to_datetime('today').strftime('%Y-%m-%d')
# Download stock data
data = yf.download(stocks, start=start_date, end=end_date)['Adj Close']
# Calculate daily returns
daily_returns = data.pct_change().dropna()
# Calculate cumulative returns
cumulative_returns = (1 + daily_returns).cumprod() - 1
# Plot the cumulative returns
plt.figure(figsize=(10, 6))
plt.plot(cumulative_returns.index, cumulative_returns['NVDA'], label='NVIDIA', linestyle='-', marker='o')
plt.plot(cumulative_returns.index, cumulative_returns['TSLA'], label='TSLA', linestyle='-', marker='o')
# Add title and labels
plt.title('NVIDIA vs TSLA Stock Returns YTD 2024')
plt.xlabel('Date')
plt.ylabel('Cumulative Returns')
plt.legend()
# Save the plot to a file
plt.savefig('NVIDIA_vs_TSLA_YTD_2024.png')
```
This script first fetches the adjusted close prices for NVIDIA and TSLA since the start of 2024, then calculates the daily returns and cumulative returns. Finally, it plots these cumulative returns, saves the plot to a file named `NVIDIA_vs_TSLA_YTD_2024.png`, and does not display the plot with `plt.show()`. The plot will be saved in the current directory.
--------------------------------------------------------------------------------
Executor:
Traceback (most recent call last):
File "/workspace/tmp_code_1fa9faaf812a102186642c2701ad5f27d58984777136fbc0f3c94e9af19e86b7.python", line 1, in <module>
import yfinance as yf
ModuleNotFoundError: No module named 'yfinance'
--------------------------------------------------------------------------------
Assistant:
It seems the `yfinance` library is not installed in your environment. You can install it using pip. Here is how you can do that:
```python
!pip install yfinance
```
After you install `yfinance`, try running the previous script again to generate the plot. If you encounter other issues or need further assistance, feel free to ask!
--------------------------------------------------------------------------------
Executor:
File "/workspace/tmp_code_b9359aeedcbd7ba9412fb5916da4628096f74824ec12d59f809e2496faca5a06.python", line 1
!pip install -qqq yfinance
^
SyntaxError: invalid syntax
--------------------------------------------------------------------------------
Assistant:
It seems like there was a misunderstanding. The `!pip install` command is used in Jupyter Notebook or other interactive Python environments and cannot be directly executed as a standalone script.
Instead, to install `yfinance` in a standard Python environment, you should run the following command in your terminal or command prompt:
```bash
pip install yfinance
```
Once `yfinance` is successfully installed, you can then run the stock plotting script provided earlier. If you are working in an environment where you can't run shell commands or install packages directly (for example, some cloud or restricted environments), you'll need to ensure that `yfinance` is included in the environment setup or consult the environment's documentation for how to manage Python packages.
--------------------------------------------------------------------------------
Executor:
--------------------------------------------------------------------------------
Assistant:
If you have any other questions or need further assistance with the script or any other issue, feel free to let me know how I can help!
From the agent's output, we can see the plot of Tesla's and Nvidia's stock returns has been created.
from IPython.display import Image
Image(filename=f"{work_dir}/NVIDIA_vs_TSLA_Stock_Returns_YTD_2024.png") # type: ignore
/var/folders/cs/b9_18p1s2rd56_s2jl65rxwc0000gn/T/tmp_9c2ylon
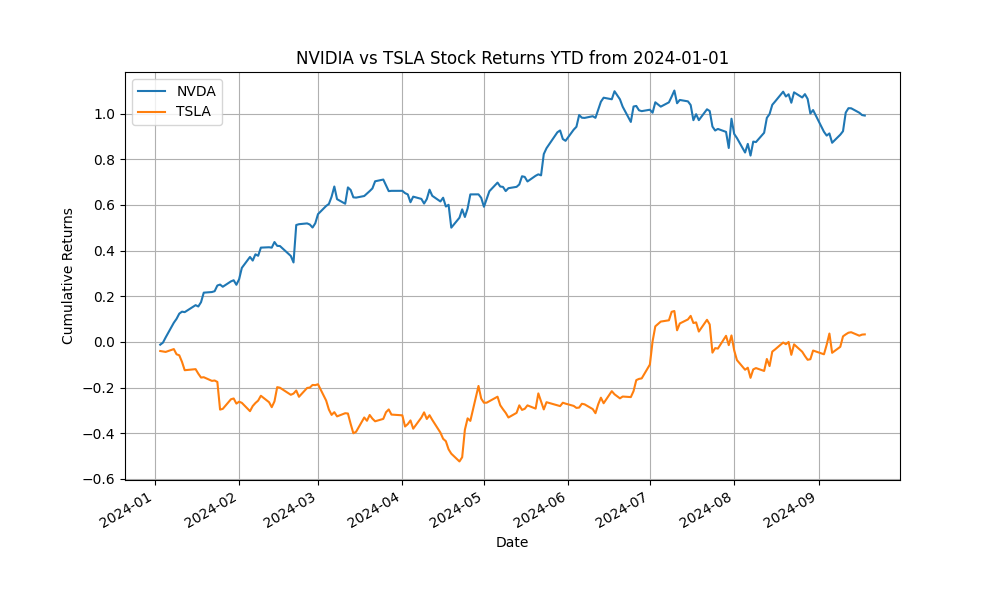
AutoGen also supports a distributed agent runtime, which can host agents running on different processes or machines, with different identities, languages and dependencies.
To learn how to use agent runtime, communication, message handling, and subscription, please continue reading the sections following this quick start.